Mixpanel
Integration
Smartlook can be easily integrated into Mixpanel
// User URL integration
Smartlook.instance.user.listeners += object : User.Listener {
override fun onUrlChanged(url: URL) {
mixpanel.getPeople().set("Smartlook User dashboard URL", url.toString())
}
}
// Session URL integration
Smartlook.instance.user.session.listeners += object : Session.Listener {
override fun onUrlChanged(url: URL) {
val eventProperties = JSONObject()
.put("URL", url.toString())
mixpanel.track("Smartlook session", eventProperties)
}
}
// User URL integration
Smartlook.getInstance().getUser().getListeners().add(url -> {
mixpanel.getPeople().set("Smartlook User dashboard URL", url);
});
// Session URL integration
Smartlook.getInstance().getUser().getSession().getListeners().add(url -> {
JSONObject eventProperties = new JSONObject();
try {
eventProperties.put("URL", url);
} catch (JSONException exception) {
}
mixpanel.track("Smartlook session URL", eventProperties);
});
NotificationCenter.default.addObserver(forName: Session.urlDidChangeNotification, object: nil, queue: nil)
{ notification in
if let smartlook = notification.object as? Smartlook,
let sessionUrl = smartlook.user.session.url {
mixpanel?.track(event: "Smartlook session URL", properties: [ "session_url": sessionUrl])
}
}
NotificationCenter.default.addObserver(forName: User.urlDidChangeNotification, object: nil, queue: nil)
{ notification in
if let smartlook = notification.object as? Smartlook {
let visitorUrl = smartlook.user.url
mixpanel?.people.set(property: "smartlook_visitor_url", to: visitorUrl)
}
}
function sessionUrlChangedCallback(sessionUrl: string) {
mixpanel.track(
"Smartlook session URL",
{"session_url": sessionUrl});
}
function userUrlChangedCallback(userUrl: string) {
mixpanel.people.set({ "smartlook_user_url": userUrl });
// identify must be called along with every instance of people.set
mixpanel.identify("sample_identifier");
}
Smartlook.instance.eventListeners.registerUserChangedListener(userUrlChangedCallback);
Smartlook.instance.eventListeners.registerSessionChangedListener(sessionUrlChangedCallback);
class CustomIntegrationListener implements IntegrationListener {
@override
void onSessionReady(String? dashboardSessionUrl) {
mixpanel.track(
"Smartlook session URL",
{"session_url": dashboardSessionUrl});
}
@override
void onVisitorReady(String? dashboardVisitorUrl) {
mixpanel.getPeople().set("smartlook_user_url", dashboardVisitorUrl);
// identify must be called along with every instance of people.set
mixpanel.identify("sample_identifier");
}
}
//Put to your init state or some place you want to register this
Smartlook.registerIntegrationListener(CustomIntegrationListener());
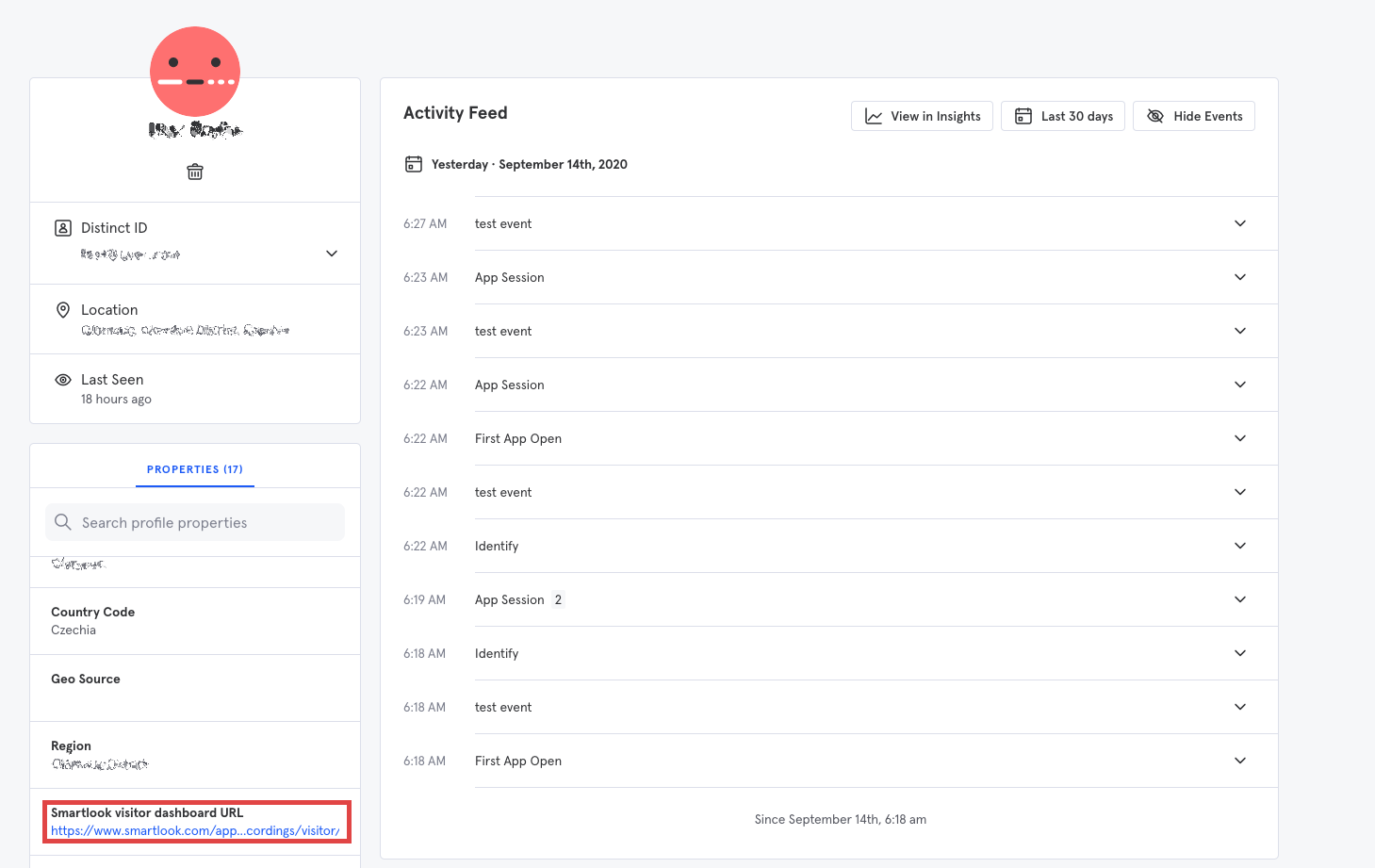
Official documentation
Full Mixpanel documentation with examples can be found on the official website.
Updated over 2 years ago