Heap
Integration
Smartlook can be easily integrated into Heap:
Smartlook.registerIntegrationListener(object : IntegrationListener {
override fun onSessionReady(dashboardSessionUrl: String) {
val props = mapOf("Session url" to dashboardSessionUrl)
Heap.track("Smartlook session URL", props)
}
override fun onVisitorReady(dashboardVisitorUrl: String) {
val props = mapOf("Smartlook visitor URL" to dashboardVisitorUrl)
Heap.addUserProperties(props)
}
})
Smartlook.registerIntegrationListener(new IntegrationListener() {
@Override
public void onSessionReady(@NotNull String dashboardSessionUrl) {
Map<String, String> props = new HashMap<>();
props.put("Session url", dashboardSessionUrl);
Heap.track("Smartlook session URL", props);
}
@Override
public void onVisitorReady(@NotNull String dashboardVisitorUrl) {
Map<String, String> props = new HashMap<>();
props.put("Smartlook visitor URL", dashboardVisitorUrl);
Heap.addUserProperties(props);
}
});
NotificationCenter.default.addObserver(forName: Session.urlDidChangeNotification, object: nil, queue: nil)
{ notification in
if let smartlook = notification.object as? Smartlook,
let sessionUrl = smartlook.user.session.url {
Heap.track("Smartlook session URL", withProperties: [ "session_url": sessionUrl.absoluteString ])
}
}
NotificationCenter.default.addObserver(forName: User.urlDidChangeNotification, object: nil, queue: nil)
{ notification in
if let smartlook = notification.object as? Smartlook {
let visitorUrl = smartlook.user.url
Heap.addUserProperties([ "smartlook_visitor_url": visitorUrl.absoluteString ])
}
}
function sessionUrlChangedCallback(sessionUrl: string) {
heap.track(
"Smartlook session URL",
{"session_url": sessionUrl});
}
function userUrlChangedCallback(userUrl: string) {
heap.addUserProperties({'smartlook_user_url': userUrl});
}
Smartlook.instance.eventListeners.registerUserChangedListener(userUrlChangedCallback);
Smartlook.instance.eventListeners.registerSessionChangedListener(sessionUrlChangedCallback);
class CustomIntegrationListener implements IntegrationListener {
@override
void onSessionReady(String? dashboardSessionUrl) {
heap.track(
identity: _identity,
event: 'dashboardSessionUrl',
properties: {
'dashboardSessionUrl': dashboardSessionUrl
},
);
}
@override
void onVisitorReady(String? dashboardVisitorUrl) {
heap.userProperties(
identity: _identity,
properties: {
'dashboardVisitorUrl': dashboardVisitorUrl
},
);
}
}
//Put to your init state or some place you want to register this
Smartlook.registerIntegrationListener(CustomIntegrationListener());
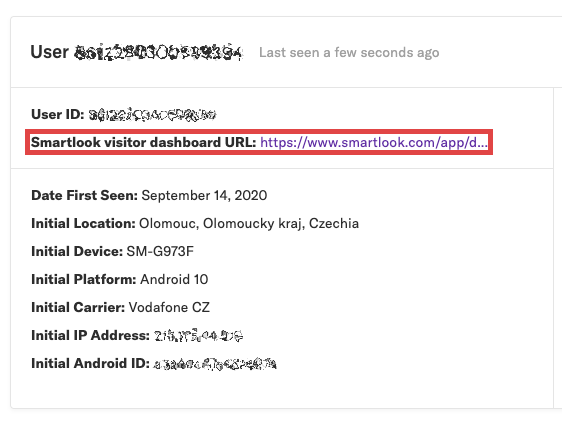
Official documentation
Full Heap API documentation can be found on the official website.
Updated about 2 years ago